How to update/force update iOS Swift app
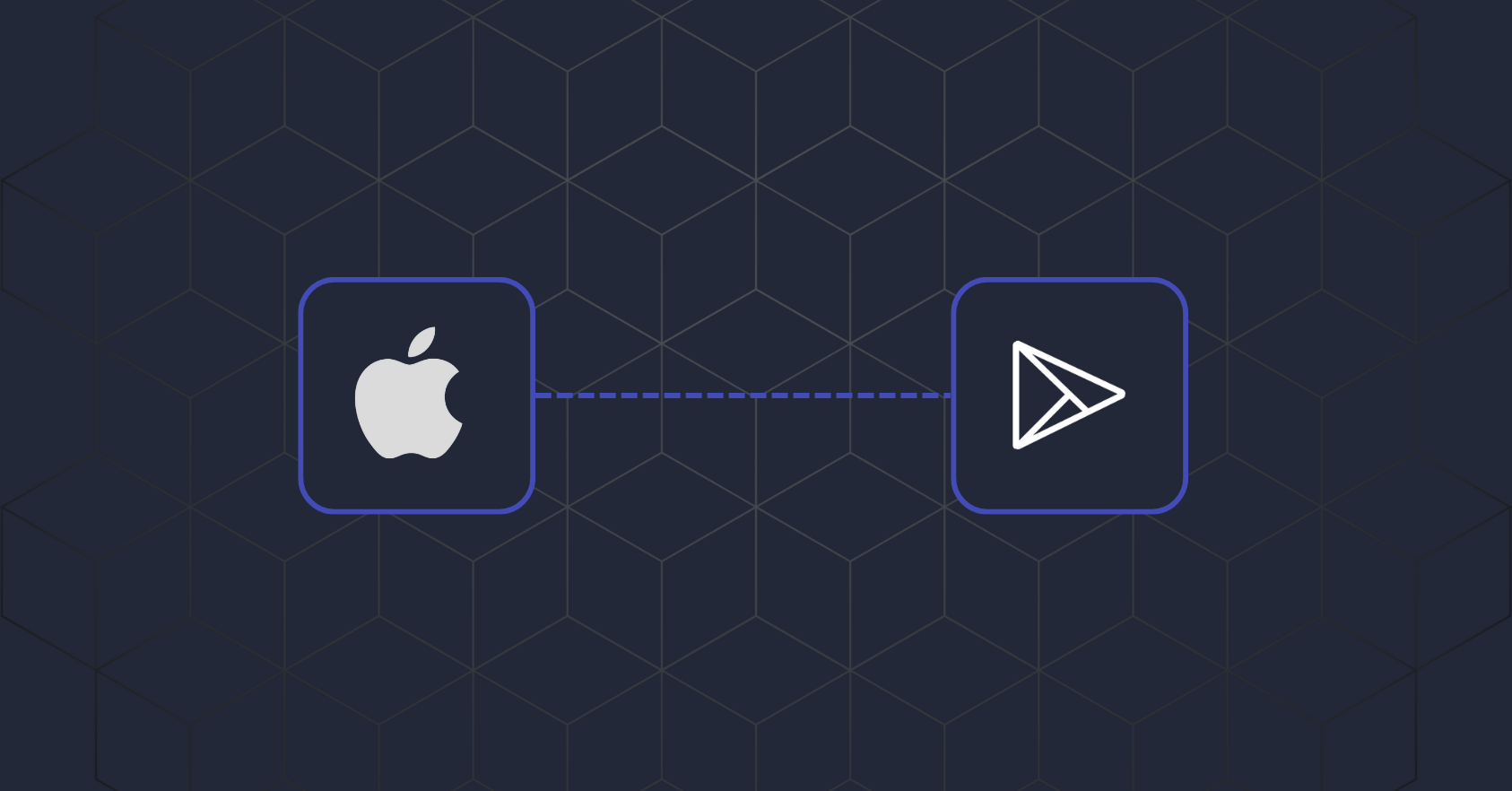
Introduction
Many times we need to force upgrade mobile apps on user’s mobile. In this blog post, we will learn how to ask users to upgrade the apps or force them to upgrade the iOS app written in Swift using App Upgrade.
1. Signup/sign in with App Upgrade:
First, let’s signup with App Upgrade. Head over to https://appupgrade.dev/signin or Signup if not already else sign in.
2. Create Project
Project is a high-level term used in App Upgrade. Project is an umbrella that you will be using to manage the versions of your app. Assuming you have done signing up and you are logged in to the app upgrade. You will be welcomed with the following screen.
Click on the +New Project button to create a new project. Provide a proper Name and Description for your project.
Click on Submit button and the project will be created.
3. Obtain the API Key:
Click on the API Key button inside the project card, and you will see the API key for your project.
Copy the API key we will need it later.
4. Integrate SDK with iOS App:
Alright, now it's time for some coding. Let's start with installing the App Upgrade iOS SDK.
Installation
1. File > Add Packages > Add Package Dependency
2. Add https://github.com/appupgrade-dev/app-upgrade-ios-sdk.git
3. Select "Up to Next Major" with 1.0.0
Use the SDK.
- Import the library
import AppUpgrade
- Update your
ContentView.swift
import SwiftUI
import AppUpgrade
struct ContentView: View {
var body: some View {
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundColor(.accentColor)
Text("Hello, world!")
}
.padding()
.onAppear() {
let appUpgrade: AppUpgrade = AppUpgrade()
let xApiKey = "ZWY0ZDhjYjgtYThmMC00NTg5LWI0NmUtMjM5OWZkNjkzMzQ5";
let appInfo = AppInfo(
appId: "String",
appName: "Wallpaper app",
appVersion: "1.0.0",
platform: "ios",
environment: "production",
appLanguage: "es" //Optional
)
// Optional
let alertDialogConfig = AlertDialogConfig(
title: "Update Require", // Optional
updateButtonTitle: "Update Now", // Optional
laterButtonTitle: "Later" // Optional
)
appUpgrade.checkForUpdates(xApiKey: xApiKey, appInfo: appInfo, alertDialogConfig: alertDialogConfig)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Let's look into the app info parameters:
- appId: App Id of your app. Know how to find appId for your app.
- appName: Your app name.
- appVersion: Current version of your app.
- platform: This could be android or ios
- environment: This could be for example development, int, production
- appLanguage: This is your app language. Example: en, es etc. This will be used to localize the update message.
5. Testing Integration
Now once we are done with the integration it's time to test whether integration works or not that is if the upgrade alert popup is showing up or not. For testing this out we will need to create a version entry.
6. Create Version
Go to Projects
and click on the Versions
button, and click on the +New Version
button.
Provide the following details:
- App Name: Name of the App
- App Version: Version of the app you want to mark for the update. For example, 1.0.0 // This is the version you want the user to force upgrade to a newer version.
- Platform: App platform example: android or iOS
- Environment: The environment in which the app is running example: development, staging, or production
- Message: An optional message which you want to show to the user when the user will be alerted of the force update.
- Force upgrade: Boolean flag if selected means this is going to be a forced upgrade. If not selected indicates it's not a forced upgrade.
After providing the value click on Submit
button and version will be created.
Reload your iOS app. SDK will check if an upgrade is required or not and show an alert popup.
7. Upgrade Alert popup
Based on the value of Force Upgrade the SDK will show a dismissable alert popup or a forced upgrade non-dismissable alert popup as shown below.
For force upgrade, only the Update button is enabled and the user cannot skip it. For recommended upgrades, the Update and Later buttons are enabled. Users can skip it.
Conclusion
We learn how to set up App Upgrade to force users to upgrade the iOS apps. To know more about App Upgrade visit: https://appupgrade.dev Also, Check out App Upgrade Documentation to understand how it works.
You can find a sample app from here app-upgrade-ios-swift-demo-app
Thanks for reading.
- If you have any queries write to us here.
- Follow us on Twitter for announcements.
- Join us on Discord.
- Subscribe on YouTube for demos and tutorials.
- Star us on Github to see what we are building.
Need help?
If you're looking for help, try our Documentation or our FAQ. If you need support please write to us at support@appupgrade.dev